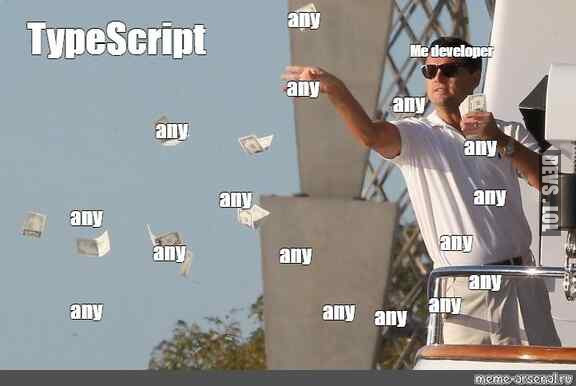
TypeScript Generics Explained !
9 October 2022
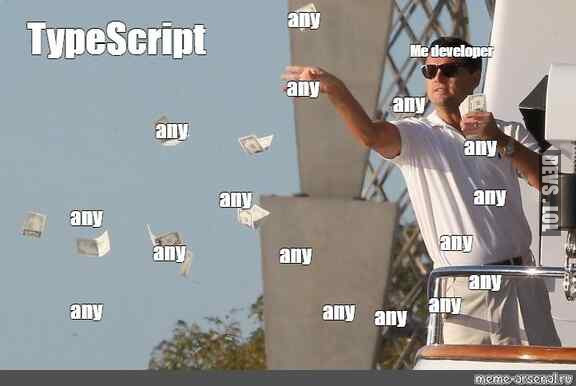
Short story about Generics in TS
Backgrund Story
if you have a function like
function printData(data: number | string | boolean | any}) {
console.log("data: ", data);
}
printData(2);
printData("hello");
printData(true);
that return data value is more than two, I think will need to refactor that, so we use Generic to solve that !
How Generics Work in TS
Generics are like variables – to be precise, type variables – that store the type (for example number, string, boolean) as a value.
So, you can solve the problem we discussed above with generics as shown below:
function printData<T>(data: T) {
console.log("data: ", data);
}
printData(2);
printData("hello");
printData(true);
You use a type variable inside angular brackets after the function name You then assign the type variable to the parameter data: T Let's explore these differences a bit more.
How to Use Generics with Interfaces
You can even use generics with interfaces. Let's see how that works with the help of a code snippet:
interface UserData<X,Y> {
name: X;
rollNo: Y;
}
const user: UserData<string, number> = {
name: "Ram",
rollNo: 1
}
In above snippet, <string, number> are passed to the interface UserData. In this way, UserData becomes a reusable interface in which any data type can be assigned depending upon the use case.